There might be scenarios where you want to make changes to your list. Lists are dynamic. You can modify their elements throughout the course your program.
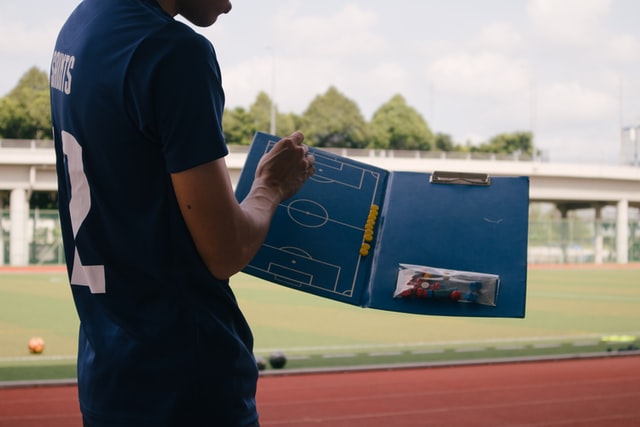
In the last article, you learned how to create a list and access its elements.
Read: How to Create a List and Access its Element
You are a football club manager. The transfer window is open and you're to make player requests to strengthen the team.
Initially, you made a request for the following players. Let's represent your request with a list.

Replacing List Elements
Discussions with Borussia Dortmund regarding Sancho have proven difficult. So you've decided to replace him with Grealish.
To change an element in a list, you have to access it and reassign the position to another element.
By counting the indexes from 0, Sancho sits on the index 2.
Run the below code in your jupyter notebook to replace Sancho.
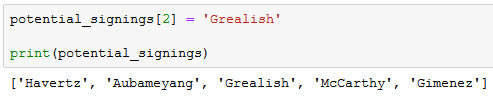
The output shows that Sancho has been replaced.
You can change any element in a list by accessing it through its index and reassigning its position to your new value.
Adding Elements to a List
The club has offloaded some players to fund the spending spree on new signings. You need to make additional requests.
You have decided to add Chilwell, Coman, Willian and Coutinho to the list.
Append Method
The append method adds an element to the end of a list.
To add the four new players to the end of the list, run the below code.
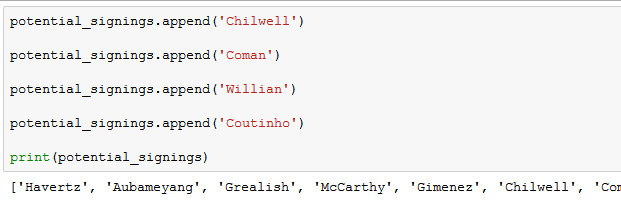
The excerpt shows that the players have been added to the end of the list in the order of the append calls
Insert Method
Important signings are put first in the list.
You would like to prioritize the signing of a new player. Instead of using the append call, you'll use the insert call to add the new player to your list.
The insert method takes two arguments(the index and value of the new element respectively).
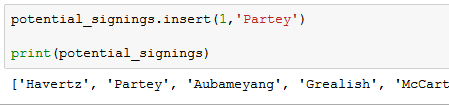
This operation shifts other values to the right.
Removing List Elements
The ask price for some of your potential signings has increased. You'll need to prune your list to make funds for them.
Del Statement
Let's remove McCarthy from the list. He sits on the index position 4 after Grealish.
You can remove an element by accessing its position as shown below.
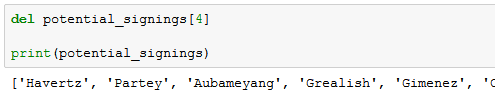
From the excerpt, McCarthy has been removed.
Pop Method
This can be used to remove the last item in a list. It also gives you access to the deleted element.
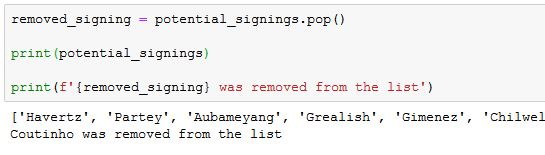
The pop method can also be used to remove an element from any position in a list. The index position of the item to be removed is included in the parentheses pop method.
Let's remove Gimenez from the list. He sits on the index position 4 after Grealish.
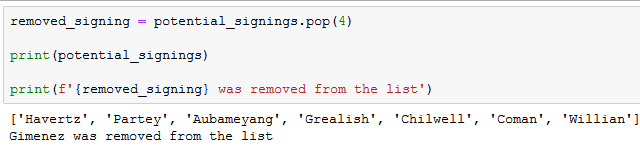
Remove Method
You might not always know the position of the element you want to delete. The remove method allows you to remove an element by its value.
Let's remove Coman from the list.

From the output, Coman has been removed from the list. The deleted item can only be accessed when the pop method is used.
The remove method deletes only the first occurrence of an element. If Coman appeared twice in a list, the first occurrence would be deleted leaving the second occurrence in the list.
To remove all occurrences of an element, you'll need to use the remove method multiple times. Loops can be useful in this scenario. You'll learn about loops in future sessions.
After several modifications on our list, let's find the total number of items in our final list. This can be done using the len function.
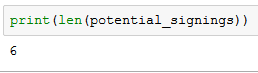
Avoid errors by ensuring you start counting index position from 0 not 1. Trying to print the element at index position 3 in a list that contains 3 elements will throw an error. The last element in this list has an index position of 2.
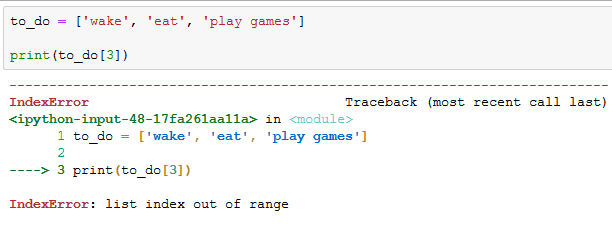
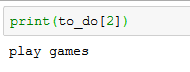
Keep in mind that you can always access the last element in a list by using an index of -1.
Summary
- List elements can be replaced by reassigning their positions to new elements
- append and insert methods can be used to add elements to a list
- The del statement, pop and remove methods can be used to remove elements from a list
- The pop method allows you to use an element after deletion
- The remove method deletes only the first occurrence of an element
No comments: